Nebeolisa Samson
FRONT-END ROADMAP
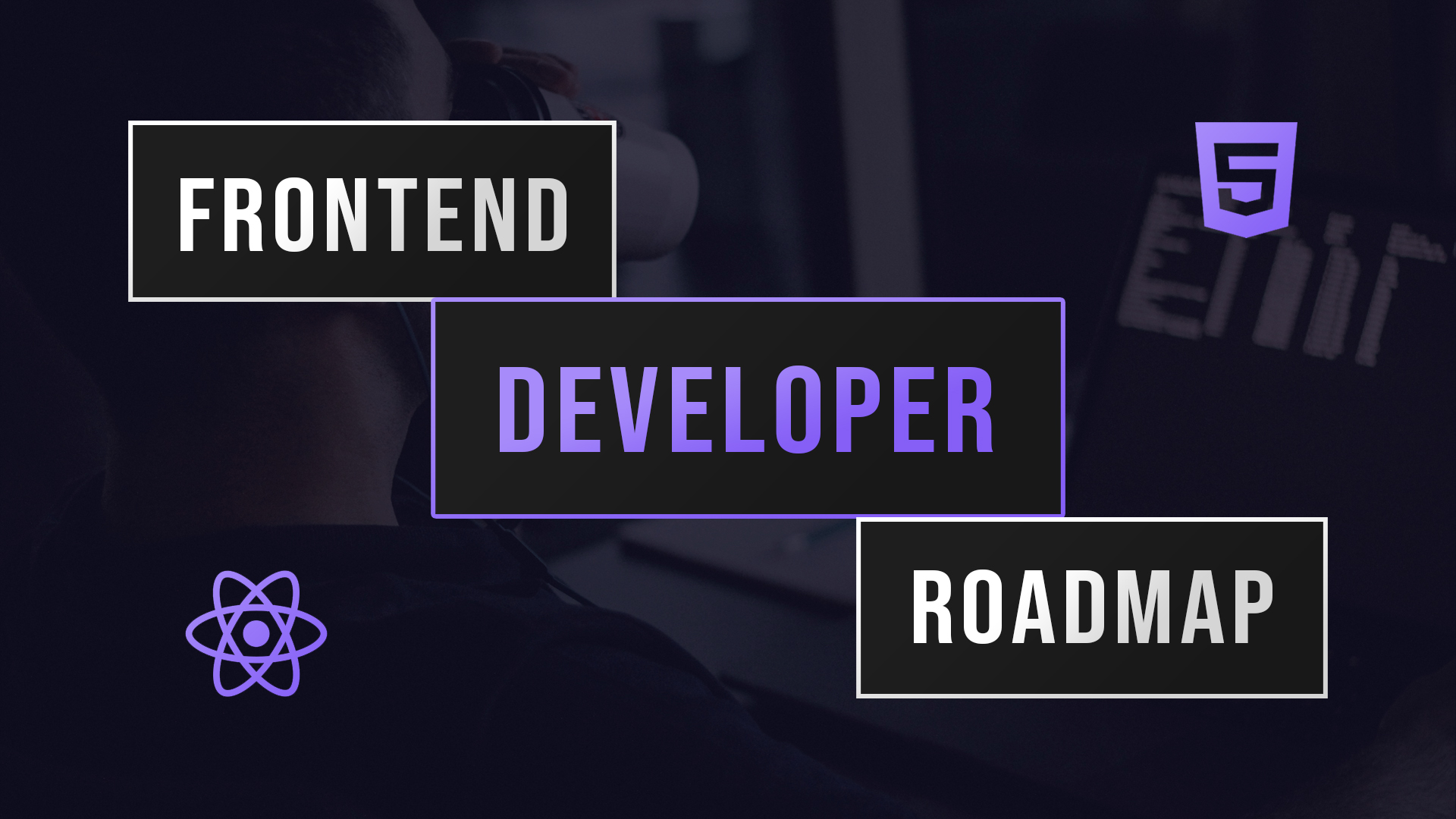
Recently, with the number of available tools, technologies and programming languages for web development, it can be overwhelming for beginners that are looking forward to becoming a front-end developer.
Front-end development is programming, focusing on the visual elements (client side) of the website or application that users will interact with. The front-end developers are people who develop the user interface of websites and applications. They are responsible for determining the structure and design of the web application, building features to improve the user experience, balancing the design and functionality, optimizing the web application for different devices, optimizing the page for speed and scalability, using different markup languages to encode web pages, maintaining brand consistency and writing reusable code.
This roadmap will guide you to the proper ways to becoming a front-end developer. I will cover the most important building blocks of the web. This guide will help you to become a modern front-end developer.
Note : This article is not a detailed explanation of the tools and technologies of a front-end developer, but just footsteps for you to follow in your journey to becoming a front-end developer.
- Basics
- Frameworks
- Preprocessor
- Package Managers
- Version Control System
- Test Libraries Or Frameworks
- Websites Deployment
- Others
- Module Bundlers
RoadMap
Learn the Basics - HTML
-
- Basics
- Forms
- Semantic HTML
- SEO Basics
HTML
HTML - HyperText Markup Language, is just the skeleton
or the foundation of any website. It is used in structuring the content of a website.
HTML makes use of a simple syntax and it is easy to learn. understanding the simple basic use
of the tags, elements and attributes of html will be a great start. Learn more about html: form attributes,
form elements, input types , input attributes, input form attributes and responsive forms.
A semantic element - clearly describes its meaning to both the browser and the developer.
Limit the use of <div>...</div>
and
<span>...</span>
in your markups. All the tags and elements
that make up html have a purpose , try and understand how or when each tag will be used for a particular
purpose and use the right elements, the right tag for the right purpose. HTML is the language you can use
to accurately tell search engines about the content of your website and how they should rank you. So a good
Search Engine Optimization (SEO) starts with a well written html.
Example of a simple html:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<header>
<nav>
<ul>
<li><a>Home</a></li>
<li><a>About</a></li>
<li><a>Service</a></li>
</ul>
</nav>
</header>
<main>
<section>
<h1>This is heading 1</h1>
</section>
<aside>
<p>I am by the side</p>
</aside>
</main>
<footer>
<p>I am the footer</p>
</footer>
</body>
</html>
In the above code you can easily see that each element has an opening <...>
and a closing </...>
tag except the <!DOCTYPE html>
which represents the document type, and helps browsers to display web pages correctly.
Learn the Basics - CSS
-
- Basics
- Selectors
- Positioning
- Box Model
- Display
- Specificity
- FlexBox
- Grid
- Media Queries
- Pseudo Elements
- Pseudo Classes
- Animations
CSS
CSS - Cascading Style Sheets describe how to display HTML elements on the screen, paper or other media. While HTML is the "skeleton" used to describe the content of on the web, CSS is the "Outer Skin" or the "coating" of the skeleton. CSS describes how the content should be formatted, which includes the looks and feel of the web page.
CSS has a simple and easy to learn syntax, made up of Selectors and declarations. Css can be a good tool for design and layout and it can be used to style the layout of multiple web pages or multiple html elements all at once. Making web pages fit and look good on all devices is also achieved with CSS. Understanding the above listed parts of CSS will help you throughout your journey of becoming a front-end developer.
Example of a simple CSS:
body {
background-color: lightblue;
}
h1 {
color: white;
text-align: center;
}
.text {
font-family: verdana;
font-size: 20px;
}
Learn the Basics - JAVASCRIPT
-
- Basics Syntax
- Dom Manipulation
- Fetch API / Ajax
- Async Await
- Event Listeners
- ES6+ JavaScript
- Promises
- Classes
- Array Methods
- Scoping
- Hoisting
- Closures
JAVASCRIPT
JAVASCRIPT - is the programming language of the web, it is the world's most popular programming language and it serves as a great first language for anyone brand new to coding. So let's take it back to human body anatomy, While HTML is the "skeleton" and the "internal organs" of a body, CSS is the outer design of the skin including the shape and placement of hair, eye, ear, toes ect. JavaScipt then adds live and functionality to the whole body and organs.
JavaScript is used to add functionality to a website, it is used to improve the interactivity of a website. It allows developers to create fast and dynamic websites. The syntax of javaScript is quite not as simple as the syntax of HTML AND CSS, but also easy to learn. Most web page animations, updates and other functionality are achieved with the use of javaScript. Understanding the concept of javascript and the above listed parts of javaScript will make your web development journey an easy one.
Example of a simple JavaScipt:
function myFunction(a, b) {
return a * b;
}
const c = myFunction(5, 5);
document.getElementById("demo").innerHTML = c;
Learn any CSS Framework
Most popular ones
-
Bootstrap
-
Tailwind
-
Materialize
-
Bulma
-
Semantic UI
-
Foundation
CSS Framework - is a ready-to-use CSS library or a standardized package files and folders of codes used in the styling of one's website. Frameworks make the life of a web developer easier. Instead of starting each project from scratch, the CSS framework provides them with tools to quickly create user interfaces that they repeat and tweak during the project. Different frameworks are suitable for different project needs, and each framework has some unique features.
With the number of available CSS frameworks to choose from, it can be overwhelming to figure out which one is most suitable for your project. The above listed CSS frameworks are the most popular frameworks at the moment and understanding any one of your choice is a wonderful step forward.
Example of a simple Bootstrap:
<div class="container-fluid text-white text-center">
<h1>My First Bootstrap</h1>
<p>
Stylings are done in the class
</p>
</div>
Bootstrap and Tailwind are the choice for many developers and companies. Try and find out the framework in need in your area or place of choice, to make collaborating with others easy for you.
Learn any CSS Preprocessor
Most popular ones
-
Sass / Scss
-
Postcss
-
Less
-
Stylus
CSS Preprocessor - is a program that lets you generate CSS from
the preprocessor's own unique syntax.
- MDN. CSS preprocessor is a scripting
language that extends CSS, the extended CSS code is compiled and returned as a regular CSS file.
We can use logic in script files, such as variables, functions, mixin, inheritance, nested inheritance,
and mathematical calculations with the help of CSS preprocessors. The CSS preprocessor enables
automatic repetitive tasks, reduces the number of errors and code expansion, creates reusable code
and ensures easy backward compatibility. As of now SASS/SCSS is the most popular preprocessor.
/* Define standard variables and values for website */
$bgcolor: lightblue;
$textcolor: darkblue;
$fontsize: 18px;
/* Use the variables */
body {
background-color: $bgcolor;
color: $textcolor;
font-size: $fontsize;
}
Learn any JavaScipt Framework
Most popular ones
-
React
-
Vue
-
Angular
-
Svelte
-
Meteor
-
Remix
JavaScript Frameworks - are context-specific structures that help you create web applications in that context. They are a collection of JavaScript code libraries that provide web developers with already written reusable code components, a universal development environment, compilers, toolsets, code libraries, APIs for everyday programming tasks. Most developers prefer these frameworks over writing javaScript codes from scratch. React, Vue, Angular are the most popular javaScript frameworks for front-end development with React.js on the lead.
React.Js - is a JavaScript library used to build interactive elements on a website in web development. It is a free open source front-end JavaScript library for building user interfaces based on UI components.
Basic things to learn in React
-
React
- Components
- Jsx
- Props
- State
- Events
- Conditional Rendering
- React Hooks
-
React UI Frameworks
- Material UI
- Ant Design
- Chakra UI
- React BootStrap
- Rebass
- Blueprint
- Semantic UI React
-
React packages
- React Router
- React Query
- Axios
- React Hook Form
- Styled Components
- Storybook
- Framer Motion
-
React state managemnet
- Redux
- Mobx
- Hookstate
- Recoil
- Akita
In the above list, number 1 and 3 is of great importance to know all the listed topics. While in number 2 and 4, it is customary to learn just one of each. After learning the above listed, Next Js, Gatsby, TypeScript, React Native, Electron are also very important to your front-end development journey.
Learn the basics of package Managers
-
NPM
-
Yarn
Package Managers or Package Management System - handles software packages, product dependencies and data distribution. It tracks the software installed on the system and allows you to easily configure softwares, install new software, upgrade the software to a newer version or delete the previously installed software. You should understand the basic commands of any of the above listed package managers.
Learn the basics of Version Control System
-
Git
-
GitHub
-
GitLab
-
Aws CodeCommit
Version Control - is a component of software configuration management that is responsible for managing and tracking changes made in the system programs, documents, large web sites, source code of Web applications, or other collections of information. The version control system is a software tool that helps developers track and manage changes to the source code over time and also revert to a previous version of the code, while providing information of why the changes were made and who made the changes. The version control system uses collaboration to enhance development speed, utilize productivity, reduce the possibility of conflicts and errors, and helps recover code in the event of an accident. Git and Github are the most popular and widely used version control systems.
Learn any Test Libraries / Frameworks
-
Jest
-
Testing Library
-
Cypress
-
Enzyme
-
Mocha
Test libraries / frameworks - Checks products and documents to determine the extent to which they meet demand or requirements. They are used to find defects in codes, measure quality and risk of web applications, build confidence, prevent bugs and ensure that the user interface of the application is working as expected. There are many levels of testing in web development, they include:
-
- Unit Testing
- Visual Regression Testing
- Acceptance Testing
- Performance Testing
- Integration Testing
- Accessibility Testing
- End-to-end Testing
- Compatibility Testing
Jest and Testing Library are the most popular Test Libraries as of the moment. As a developer testing your applications to ensure usability and functionality is as important as creating the application itself. Learn any one of the above listed Testing libraries.
Learn any website Deployment tool
Some free popular service
-
Netlify
-
Vercel
-
Firebase
-
Github Pages
-
Heroku
Website Deployment - is the process of moving your locally coded website or web applications from the local system to the internet( host (server)), so that users can visit and interact with it. Understanding the basics of any of the above listed hosting tools, is of great importance for your web application deployment. Netlify and Vercel are the two most popular website deployment tools.
Other important Topics
- PWA
- Web Sockets
- CORS
- JSON
- RESTful API
- GraphQL API
- Basic Security
All front-end developers tend to often build websites that interact with APIs, as most of the web applications are built and improved using web browser APIs. APIs are used to enhance reliability, capabilities and integration to reach anyone, anywhere, on any device with just one codebase. Connecting to the internet can cause major security issues, so it is of great importance that a front-end developer understands the importance of web security and communication protocols. Thus, learning the above listed important topics will also help you in your journey of becoming a frontend developer.
Optional Things to learn
Module Bundlers
-
Webpack
-
Parcel
-
SnowPack
-
Rollup.js
-
Gulp
Summary
The Tools for front-end development or web development are always changing, so it is of great importance for you to always be up-to-date and update your skills. I hope this article helped you in understanding the steps to becoming a front-end developer. Good luck on your journey!
Note: You don't need to learn all the things mentioned in this roadmap to become a front-end developer or get a job as a front-end developer, and it's not a must to follow this roadmap in the exact order, but it is ideal to do so. There is no end to learning in web development, there's always something to learn. So never stop learning!
I create awesome websites you will love. Working with you from start till end to make sure your goals are met and you are happy with the outcome is my number one priority.